<script type="text/javascript">
console.log(history);
</script>
<a href="myLocation.jsp?myName=ksmart">myLocation.jsp이동</a><br>
<button type="button" onclick="move()">myLocation.jsp이동</button>
<script type="text/javascript">
function move(){
location.href = 'myLocation.jsp?myName=ksmart';
}
</script>
1. 브라우저 객체 모델
1) window
- window 키워드는 생략이 가능하다.
▼ script 코드 부분
<script type="text/javascript">
console.log(window, this);
</script>
▼ 결과 확인

1. open, close
- open : 새창 열기
- close : 창 닫기
▼ script 코드 부분
<!-- on 접두사를 가진 속성들은 자바스크립트 코드삽입이 되며, 사용자가 해당 행위 했을때 스크립트가 작동 -->
<button type="button" onclick="myPageOpen()"> 새창 열기 </button>
<script type="text/javascript">
/*
open('주소', '창 이름', '옵션(새창의 사이즈 및 탭과 스크롤 노출 여부 설정)')
3번째 인수에 대한 옵션
width -> 창 가로 사이즈
hegiht -> 창 세로 크기
left -> 새창 띄울 절대값 가로 위치
top -> 새창 띄울 절대값 세로 위치
scrollbars -> 스크롤 노출 여부
*/
function myPageOpen(){
//- 절대 경로, 상대 경로
/* open(
'https://naver.com',
'naver',
'width=300, height=400, left=200, top=200, scrollbars=no'
); */
//팝업 닫기에서 저장된 myPopupClose의 값이 Y가 아니라면
if(getCookie('myPopupClose') != 'Y'){
open(
'mypopup.html',
'내 팝업',
'width=300, height=400, left=200, top=200, scrollbars=no'
);
}
}
</script>
▼ 팝업 내부 코드선언 부분(다른 html파일로 작성하였다.)
<h1>내 팝업</h1>
- 환영 합니다.
<label>
<input type="checkbox" id="oneDay"> 오늘 하루 동안 안보기
</label>
<button type="button" onclick="myPopupClose();">창 닫기</button>
<!-- 쿠키 공통 함수가 저장된 외부파일 불러오기 -->
<script type="text/javascript" src="cookie.js"></script>
<script type="text/javascript">
function myPopupClose(){
/*
document.querySelector -> css 선택자로 html 요소 선택
checked -> 체크가 되어 있다면 true 안되어 있다면 false반환
*/
let isCheck = document.querySelector('#oneDay').checked;
if(isCheck){ //체크가 되어 있다면 쿠키 값 저장
//myPopupClose=Y 하루 유지
setCookie('myPopupClose', 'Y', 1);
}
close();
}
</script>
▼ 결과확인
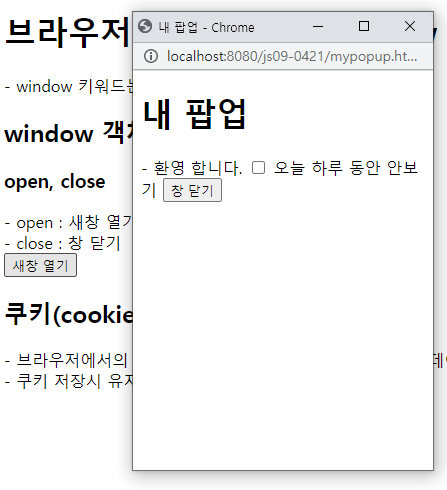
>> 체크박스 선택 후 창닫기 버튼을 클릭하면 쿠키에 값이 저장되기때문에 다시 새창열기를 시도해도 창이 열리지 않는다.(쿠키 삭제시 다시 열린다.)
2. alert
- 사용자에게 경고창을 나타내고 테이터를 보여주는 창 출력
- alert창 뜬 후에 확인 버튼이 눌러져야 아래의 코드가 실행된다.
<script type="text/javascript">
alert('안녕하세요.');
console.log('alert 종료');
</script>
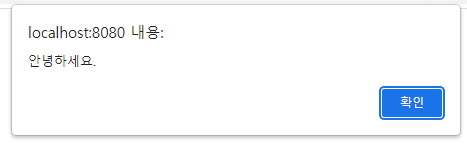
3. confirm
- 사용자에게 진행 여부를 확인하는 창
- 확인을 눌렀을 경우 리턴값 true, 취소를 눌렀을 경우 false 반환
<script type="text/javascript">
if(confirm('정말 저장하시겠습니까?')){
//확인 눌렀을 경우 출력
console.log('저장되었습니다.');
}else{
//취소 눌렀을 경우 출력
console.log('저장 취소 되었습니다.');
}
</script>
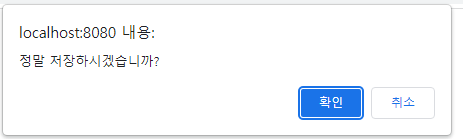
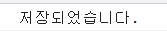

4. setInterval, clearInterval
- setInterval -> 1번째 인수 함수, 2번째 인수 시간 : 2번째 인수 시간 주기로 1번째 인수 함수 실행
- clearInterval -> 1번째 인수 setInterval 이벤트 번호 : 동작하고있는 setInterval 이벤트 동작 중지
<button type="button" onclick="myClearInterval()">반복 중지</button>
<script type="text/javascript">
var cnt = 0;
var intervalNumber = setInterval(function(){
cnt++;
console.log(cnt);
if(cnt == 10){
clearInterval(intervalNumber);
}
}, 1000);
function myClearInterval(){
if(confirm('동작을 중지 하시겠습니까?')){
clearInterval(intervalNumber);
}
}
</script>
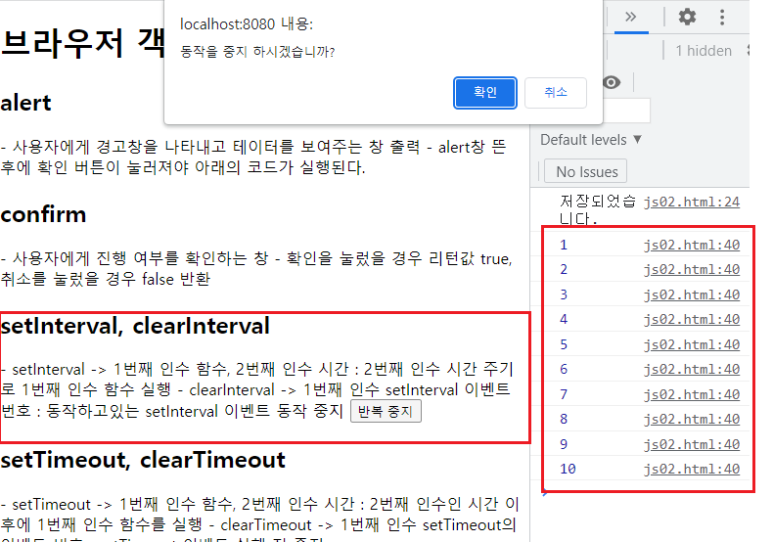
>>> 값이 1초당 1씩증가하여 10이될경우 자동으로 중단된다. 반복중지 버튼 클릭시 즉시중단된다.
5. setTimeout, clearTimeout
- setTimeout -> 1번째 인수 함수, 2번째 인수 시간 : 2번째 인수인 시간 이후에 1번째 인수 함수를 실행
- clearTimeout -> 1번째 인수 setTimeout의 이벤트 번호 : setTimeout 이벤트 실행 전 중지
<button type="button" onclick="myClearTimeout()">창 닫기 중지</button>
<script type="text/javascript">
var timeoutNumber = setTimeout(function(){
close();
}, 5000);
function myClearTimeout(){
if(confirm('창 닫기를 중지 하시겠습니까?')){
clearTimeout(timeoutNumber);
}
}
</script>
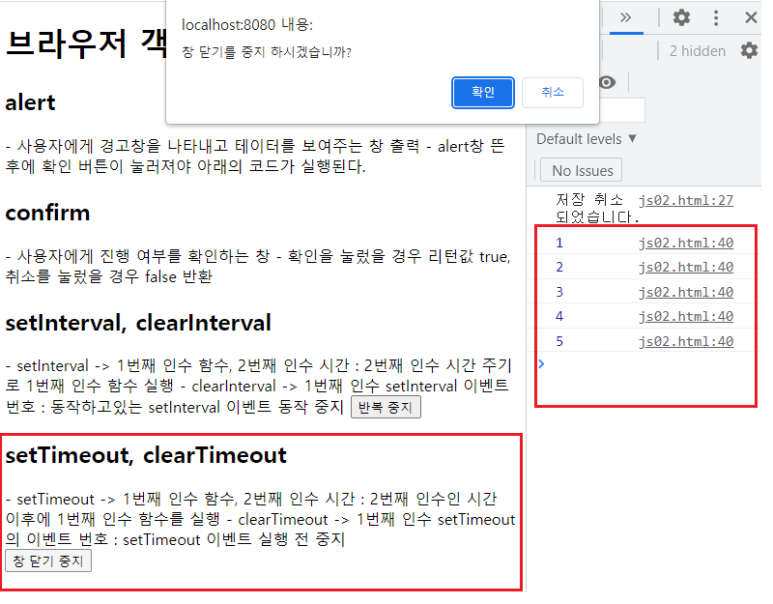
>>> 값이 1초당 1씩증가하여 5가되면 창이 자동종료된다. 창 닫기중지 버튼 클릭시 창 종료가 중단된다.
'javascript' 카테고리의 다른 글
Document API - 객체 검색 (0) | 2023.05.15 |
---|---|
html 요소 이벤트, on-- 속성, form 객체 (0) | 2023.05.15 |
쿠키(cookie) (0) | 2023.05.15 |
내장객체 (0) | 2023.05.15 |
객체생성자함수와 상속(prototype) (0) | 2023.05.15 |